Getting Started with Javascript
By going through these steps you’ll enable Facebook sharing in your game and set it up so that the posts can be edited and A/B tested without making any code changes.
The posts you will be making at the end of this QuickStart will be Open Graph Stories that look like this:
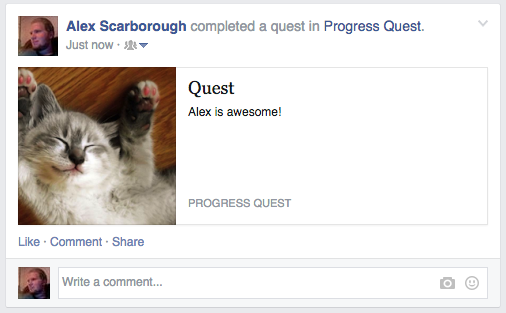
Of course, our goal is to set this up so that your creative team can replace the art and text with stuff that isn’t programmer art. So, let’s get started.
Before we begin
Before we start the SDK integration you’ll need to complete some initial dashboard setup. For that you will need to have:
A game or a prototype. If you don’t have either, you can use our sample app to test it out.
A Facebook App ID (What’s this?)
If you already set up your app and created a story, you can skip ahead to the SDK.
Getting Teak set up
First you’ll need to add your Facebook App ID to Teak.
To do this:
1. Copy your Facebook ID and Secret from the Facebook developer dashboard.
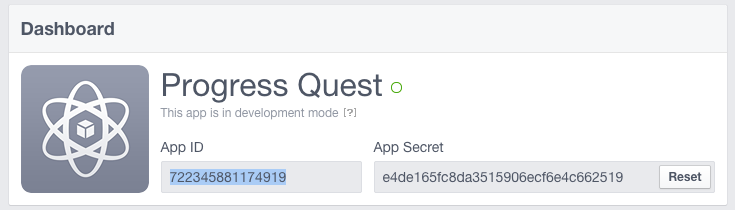
2. Enter your Facebook ID and Secret into the Teak new app page.
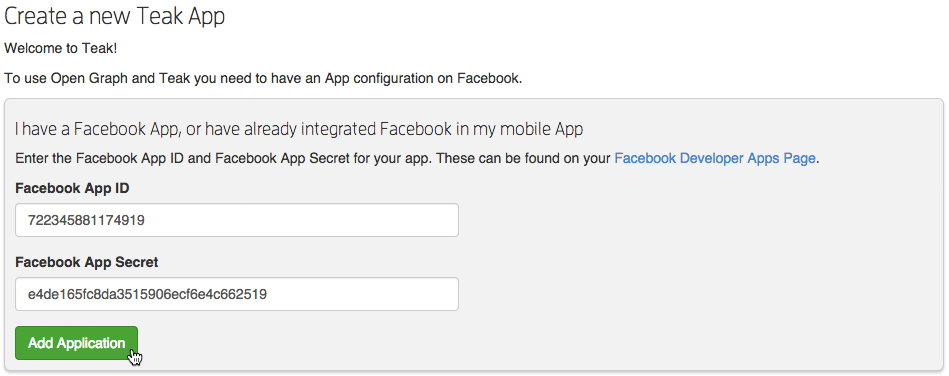
3. Click ‘Add Application’.
Alright, we are on our way! Now, let’s create some content to post to Facebook.
Create your first story
After you’ve added your app to Teak, we need to create a story to share to Facebook. Think of an action that happens in your game or prototype that players will want to share.
The format is a noun and a verb pair. So, for example, some common actions are ‘Reach a New Level’ or ‘Win a Jackpot’.
For our sample we are using ‘complete a quest’.

Now that we have a story, it’s time to create an instance of that story. The instance is the post which will be shared to Facebook, and represents a specific thing in your game.
If your story is about beating levels, each instance will be the levels that you can beat. For our story of ‘complete a quest,’ the instance is a specific quest in our game that the user can complete.
I could have created a clever story about the “The Kitten Quest,” but instead I went with “Quest.” I’ll make marketing give it a better name later.
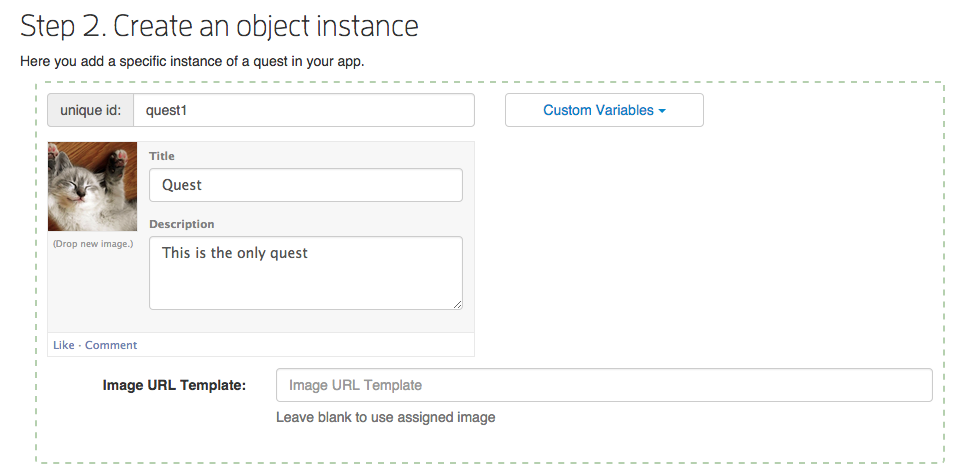
The important thing here is the unique identifier. This is how you will reference this instance in your code.
You can see I have named it “quest1” in my example. All the other things that I put in are to control how the post will appear on Facebook.
Now that we have a story, let’s test do a test post.
See your post on Facebook
Click the ‘Login with Facebook’ button and Teak will set everything up and make your first test post. Click ‘View on Facebook’ to see how your post looks on Facebook.
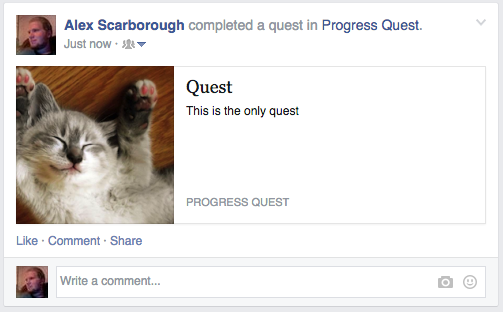
Now you’re ready to integrate the Teak SDK and make the same post directly from your game!
Posting from your Game
Okay, now that we have some content to post, we need to hook it up to our game so that our players can post from our game.
Add the Teak SDK
The Teak Javascript SDK is loaded asynchronously so it has no impact on your game’s load time. To add the Javascript SDK, insert the following code immediately after the opening <body> tag:
<script>
(function(){window.teak=window.teak||[];window.teak.methods=["init","on","asyncInit","identify","trackEvent","postAction","postAchievement","postHighScore","canMakeFeedPost","popupFeedPost","reportNotificationClick","reportFeedClick","sendRequest","acceptRequest","loadInboxData"];window.teak.factory=function(e){return function(){var t=Array.prototype.slice.call(arguments);t.unshift(e);window.teak.push(t);return window.teak}};for(var e=0;e<window.teak.methods.length;e++){var t=window.teak.methods[e];if(!window.teak[t]){window.teak[t]=window.teak.factory(t)}}var n=document.createElement("script");n.type="text/javascript";n.async=true;n.src="//d2h7sc2qwu171k.cloudfront.net/teak.min.js";var r=document.getElementsByTagName("script")[0];r.parentNode.insertBefore(n,r)})()
</script>
See this in the JS sample app.
Initialize the SDK
Before anything can be done the SDK must be initialized. You do this with the ‘init’ call, like so:
<script>
window.teak.init("YOUR_APP_ID", "YOUR_API_KEY");
</script>
YOUR_APP_ID and YOUR_API_KEY can be retrieved from your game’s Settings page on Teak.
See this in the JS sample app.
Add Facebook Login
If you don’t already have Facebook Login in your game, you will need to add it. In Javascript this can be done with the following code:
<script>
window.fbAsyncInit = function() {
FB.init({
appId : "YOUR_APP_ID",
status : true,
xfbml : true,
version : "v2.1"
});
FB.getLoginStatus(function(response) {
if (response.status === 'connected') {
// User is logged in
}
else if (response.status === 'not_authorized') {
// Do redirect to authorize the app
window.top.location = "https://www.facebook.com/dialog/oauth?client_id=YOUR_APP_ID&redirect_uri=https://apps.facebook.com/YOUR_APP_ID/&scope=email,publish_actions";
}
else {
alert("User not logged in to Facebook");
}
});
};
(function(d, s, id){
var js, fjs = d.getElementsByTagName(s)[0];
if (d.getElementById(id)) {return;}
js = d.createElement(s); js.id = id;
js.src = "//connect.facebook.net/en_US/sdk.js";
fjs.parentNode.insertBefore(js, fjs);
}(document, 'script', 'facebook-jssdk'));
</script>
In order for Teak to function you must request the publish_actions permission. If you do not request the publish_actions permission, your users will not be able to make posts from your game.
See this in the JS sample app.
Identify your Users
After the user has logged in with Facebook you’ll need to identify them to Teak. This will tell Teak to start a session for that player, and all subsequent Teak SDK calls will act on the identified user. You identify users with the ‘identify’ SDK call.
FB.getLoginStatus(function(response) {
if (response.status === 'connected') {
window.teak.identify(response.authResponse.userID, response.authResponse.accessToken);
}
});
See this in the JS sample app.
Make a Post
You are now ready to post to Facebook from your game! To make a post you will need to
- Add a checkbox allowing the user to not post, or a button for the user to click to trigger the post
- Call window.teak.postAction when the user clicks the button or if the checkbox is checked
If you are following our sample, here is how you would make the postAction call:
// postAction(actionType, objectType, instanceIdentifier, parameters);
window.teak.postAction("complete", "quest", "quest1", {"fb:explicitly_shared": true});
For your own game, use the action, object, and identifier which you created during the dashboard setup.
See this in the JS sample app.
See your post on Facebook
Now that you’ve made your post you can see it on Facebook. Just go to your activity log to see it.